JavaScript, for web apps
Since Netscape created JavaScript to program dynamic HTML pages, applications have continued to expand.
Its main function and scripting a HTML page or a graphical interface (see Qt, XAML, etc ...). This allows the exchange of data with the server through Ajax, WebSockets, WebRTC.
But it is used also to enable scripting
in some applications (Photoshop uses JavaScript) and even for system programming!
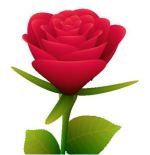
JavaScript was successively called Moka, then LiveScript and finally in agreement with Sun, JavaScript.
JScript is a compatible version implemented by Microsoft in 1996, for Windows
only.
Its conception is a mixture of C and Self according to the author, Brendan Eich, who was also had a background in Scheme The language has many flaws, but it is innovative and flexible, and although it is more than 20 years, it offers more dynamism than most newer languages, such as Dart. And implementers are working to suppress all theses flaws.
To complement ECMAScript 6 which makes JavaScript a full-fledged application language, Google has proposed in 2015 the SoundScript mode which also provides typed variables and different accelerations at runtime. But this project was abandoned in February 2016 and the team was reassigned to Dart. Typed variables are however planned in a future version of ECMAScript.
Short history of the language
- 1995. Creation by Brendan Eich. (The same year as Java).
- 1996. Implementation in the Netscape 2 browser.
- 1996. Microsoft implements JScript, a compatible language, in Internet Explorer 3.
- 1997. First ECMAScript standard.
- 1999. ECMAScript 3.
- 2009. ECMAScript 5.
- 2011. Google wants to replace JavaScript with Dart, but will reconsider in 2015.
- 2012. Microsoft launches TypeScript, a superset of JS with classes compiled to ECMAScript 5.
- 2013. Mozilla defines Asm.js, a subset of JS usable as bytecode.
- 2015. Google creates SoundScript, a strict version that facilitates optimizations for compilers.
- 2015. ECMAScript 6. Many elements are already implemented in browsers.
An ingenious and innovative language
The format described here is ECMAScript 1.5, the standard defined by the ECMA group in 1999. More recent version exists, but not supported by all browsers.
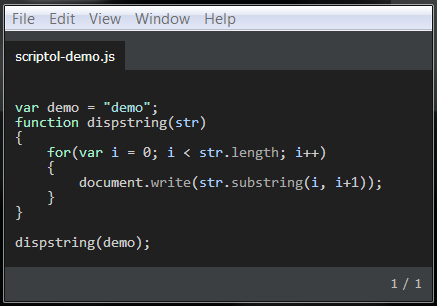
Example of code: loop on a string
- The syntax of the language is similar to that of Java or C.
- Dynamic variables. Declared as var without type, data of different types can be assigned to a same variable. Firefox in the version 9 introduced type inference which makes the compiler can process variables as typed and speed up the processing considerably.
- Object oriented, uses elements of the page as objects. Dynamic objects.
- Prototypal inheritance.
- No file management nor input-output functions (but dialogue boxes).
Local file access is provided in HTML 5 or Node.js. - Control of the browser.
- Events.
- Dynamic and associative arrays.
- Primitive types (but undeclared) are: boolean, string, number, date, array.
- Build-in Date, Math, RegExp objects.
- A for..in construct allows to scan an associative array.
- Functions are declared with the function keyword, without return type, which is optional. They are called first-class, ie, they can be used as components of the language, then be assigned to variables, embedded into structures (such as methods).
- Operators are those of ALGOL 68/C plus strict ones: === and !==, for comparing both values and types.
- Elements of a web page are accessed as a hierarchy of objects, including those of the Document Object Model (DOM, a standard by the W3C): document, window, form, table, etc...
- The hoisting: what is declared within a scope, is moved at the top. For example, if you declare a variable somewhere in a function, it will actually be declared in the beginning. Functions also are accessible anywhere in a script because they are declared at the beginning of the script.
Some flaws of the language...
- You can not replace a character in a string.
- The addition of a string and a number is not symmetrical. In one case it returns a string, in the other a number.
- Boolean comparisons are not consistent.
- The argument of the array constructor can be either the number of items or a list of elements.
ECMAScript 7 for bigger and modular apps
The new versions of JavaScript while preserving the compatibility of this language make it safer to develop huge applications. You can write ES6, ES7 code and translate for compatibility with any browser by a tool like TypeScript (Microsoft), Traceur (Google) or Babel.
- let and const.
They are used to declare a variable or a constant, either globally or locally. Let allows to declare a variable visible only within a block of instruction while with var, even declared in a block it is visible throughout the whole body of the function.
let is similar to with, with a gain in performance as it avoids creating a dynamic object as with does. - Map.
Or associative array where keys are objects, but not taken into account by the garbage collector. The list of keys may be returned. Values are retrieved by get, and pair key/value added by the set method. - WeakMap.
This is an associative array where keys can not be numeric, intendend to associate data to a list of objects.
Keys are garbage-collected objects and it is not possible to obtain a list of keys without to build it itself. - Set.
Simple mist of values, like an array, but the values must be unique.
Map and WeakMap have a set method, which is not capitalized. The Set collection has only two methods, add and has. - WeekSet
Array of unique objects. - Proxy.
Another source of confusion, this is a meta-object, or virtualized object. The behavior must be fully defined by the programmer. Creating a proxy to another object, allow to intercept the properties by the proxy, for purposes of supervision, or debugging. - Module.
By encapsulating the content of a file into module {}, we can import it as a whole, or in parts for functions preceded by the reserved word export. It works like in Node.js. This is accompanied by the Loader API to load modules. - Class .. extends
Classes with inheritance are added beside current objects. Attributes may only be defined inside the constructor. Section 14.5. - Array.from
Suppresses the current default of the new Array() constructor. With from we assign an argument that is an array, and a length property give the size of the array to create. - For .. of
Enumerates the values of properties of an associative array. for(var v of x) replaces:
for(var k in x) { var v = x[k]; }
- You may also get the pairs key/value with: for(var [k,v] of x.
- Function * and yield
This type of function is a coroutine. The yield keyword indicates the goal to marks when it terminates. This replaces callbacks in Node.js while maintaining asynchronous mode. (Chapter 13.4 of the specification). - Functions will also have default values for omitted arguments.
- Promise. An object that is a place holder for the result of a deferred or asynchronous action.
- Async/await. Make synchronous what is asynchronous.
- Template (string interpolation)
A new type of literal string can be written on several lines and incorporate variables. - Arrow. New form for a lambda function which may be now written on two ways like that:
function(a, b) { return a + b }
(a, b) => a + b - The Shared Array Buffer and Atomics are basics for concurrent programming.
- The language becomes more functional with Object.value and Object.entries that allow you to enumerate the elements of an array or the properties of an object.
To see what is implemented, view the test of implementation of new JavaScript features with this browser.
To enable some of these features in Chrome, you must type chrome: flags and Enable Experimental JavaScript.
Reference: ECMA Standard Specification for 7th Edition. 2016.
What JavaScript interpreter for each browser?
Browsers compete on JavaScript engines like on renderers... But there are now JIT compilers rather than interpreters...
- V8. The compiler of Chrome and Node.js.
- JavaScriptCore. That of WebKit and Safari. It also has other trade names: Nitro, SquirrelFish. But it's the same engine.
- SpiderMonkey. In Firefox.
- IonMonkey. In Firefox (since 18), a special JIT that runs only on Asm.js code. The faster in May 2014.
Nashorn produces bytecode for the JVM. Not integrated to any major browser.
A system language?
With the advent of JavaScript compilers, operating both in the browser or as a standalone tool, new uses are emerging for this C-like language but even freer, and with an automatic memory management, a sandbox.
- JSLinux.
This project allows you to run Linux in the browser. Program may be edited, compiled and ran at the command line. - LLVM.js.
Itt is possible to compile any LLVM language (C++, Julia) to JavaScript: the LLVM bitcode is translated to JavaScript by LLVM.js.
JavaScript is it slow on mobile?
The controversy was started by an article by Drew Crowford that JavaScript is too slow because mobiles have little memory compared to desktop computers.
But other language specialists do not agree and believe that the culprit is not the language, it is the DOM. We know that DOM is slow, which is why some frameworks like Angular have implemented data-binding that allows to update the content without going through it.
The writer adds that in fact, JavaScript can be faster than other languages like Objective-C (used on iOS) in exchanging messages because it is something that works better with a JIT than with a static compilation.
Finally, the author attributes the speed of native iOS apps to the fact that the screen is one size, unlike Android or other systems.
Even if the language is relatively easy to learn, it has dark spots, so that there are books specializing in the good parts of the language, others on complex issues.
- Tutorial of the JavaScript language.
Understanding the functions with interactive demos. Mainly the good parts... - JSON
A data format in JavaScript syntax. - ECMAScript
The official 1999 definition of the language by ECMA. - The future of JavaScript
By Brendan Eich, a diaporama describing the development of the language. The goal is to make JS suitable for applications, libraries and to replace a virtual machine.
- TypeScript. (Microsoft) Written to extend JavaScript, uses the same syntax.
- Traceur. ECMAScript 6 to 5 compiler by Google.
- Scriptol. Full support of the Scriptol language with reactive programming.
- Emscriptem. Compile LLVM bytecode to JavaScript. So any language compiled by LLVM such as C++ may be converted to JavaScript and run in the browser. Go may be converted with Gopherjs.
- Babel. (Formerly 6to5) Allows you to write ES6 or ES7 code with classes, getters, default argument values, it will be compiled to classic JS.
You can develop a JavaScript app in an IDE as LighTable (see image above), Eclipse, NetBeans or Aptana, or a simple script with any editor.
- Aptana Studio
IDE for JavaScript, HTML and CSS. It is based on Eclipse, but provides a more conventional interface. You can add plugins for other languages like PHP.
It offers also an HTML 5 boilerplate, and an interface to Git. - Karma.
By Google, test the code for all browsers, through the editor (the former name was 'Testacular' that was not appreciated by all users). - JSLint
Online tool to test your JavaScript code and find errors (by JSON creator). - Duktape
JavaScript engine to embed in a C or C++ application, possibility to add a scripting tool or dynamic code. Just add duktape.c and duktape.h to the list of sources of the project. You can then call JS 5 functions from C and vice versa. - List of languages that compile to JavaScript
The list is huge and includes Go, Dart, Python, Ruby, Java, Scala, Basic, Pascal, C and C++ through Emscriptem.
- Node.js.
You may use the V8 compiler at command line with Node (see the JavaScript section on this site) and use NPM libraries.
Electron is based on Node and the Chrome browser to build local apps.
It is strongly recommanded to use an Ajax framework or an HTML 5 library to build a serious application. There are also frameworks for mobile apps.
- River Trail.
Extension to JavaScript provided as a plugin that allows parallel programming.
Used with WebGL for example, it allows to handle thousands of objects simultanously. - HTML 5 frameworks
JavaScript libraries using Canvas. - JavaScript game engines
They do half the development. - Algorithms and data structures.
Compilation on GitHub.
JavaScript is now the language of applications on Linux
Linux
Obviously this causes protests because each developer has his favorite language (C, Python, Ruby, etc.), he would have wanted selected for this role.
The other reference environment, KDE is based on Qt, which also uses JavaScript for the interface and C++ for applications. However, the Plasma interface, which is equivalent to Metro for Linux, allows the rapid creation of JavaScript applications with the IDE Plasmate.